Step 1 - Create project with C++ application and print to debug console
In this step you first create a new Kanzi Studio project with a C++ application. You then add the code to the C++ application to print Hello world! to the Kanzi debug console.
The <KanziWorkspace>/Tutorials/Hello world/Completed directory contains the completed project of this tutorial.
Create a project with C++ application and print to debug console
To create a project with a C++ application and print to the Kanzi debug console:
-
In Kanzi Studio Quick Start window click New Project:
- Choose the name and location for your project.
For example, name your project Hello world. - Set the Template to Application.
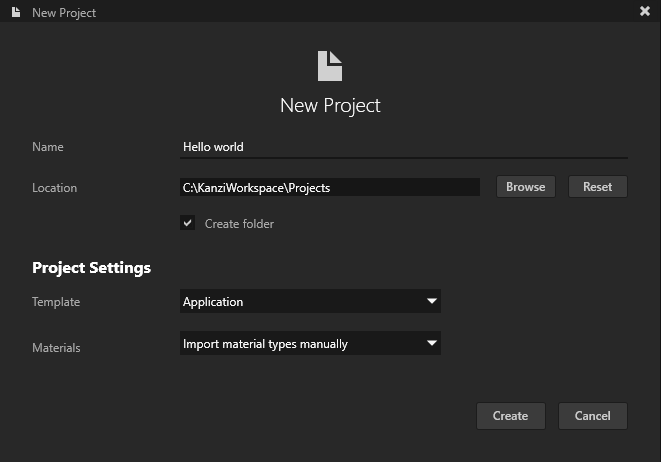
Kanzi creates a Kanzi Studio project in <KanziWorkspace>/Projects/<ProjectName>/Tool_project directory and the structure for the Visual Studio solution for your project in <KanziWorkspace>/Projects/<ProjectName>/Application:
- bin directory contains the binaries and configuration files of your project.
- configs directory contains the configuration files for different platforms. By default Kanzi creates configuration files for several different platforms. The recommended platform for developing Kanzi applications is Microsoft Visual Studio. See Deploying Kanzi applications.
- src contains the source code for your project.
- In Kanzi Studio select > Export > Export KZB.
Kanzi Studio creates the kzb file and configuration files from your Kanzi Studio project. Kanzi Studio stores the exported files in the <ProjectName>/Application/bin directory or the location you specify in the Binary Export Directory property in > . The kzb file contains all nodes and resources from your Kanzi Studio project, except the resources you mark in a localization table as locale packs.
When you run your Kanzi application from Visual Studio, your application loads the kzb file and configuration files.
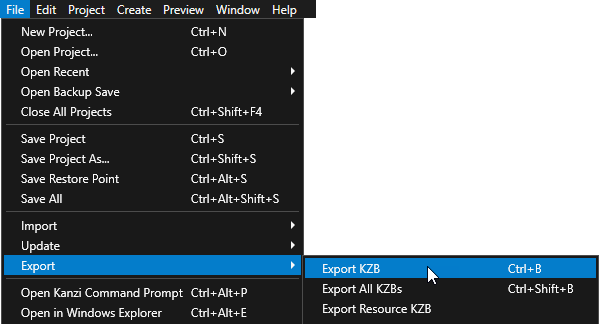
- In Visual Studio open the Visual Studio solution for your application located in Application/configs/platforms/win32.
For example, if you named your Kanzi Studio project Hello world, the Visual Studio solution is called Hello_world.sln.
NOTEIf you open the tutorial solution in Visual Studio 2017, when asked to retarget the project to the latest Microsoft toolset, click Cancel.
TIPTo open the directory of a Kanzi Studio project from Kanzi Studio, select > Open in Windows Explorer.
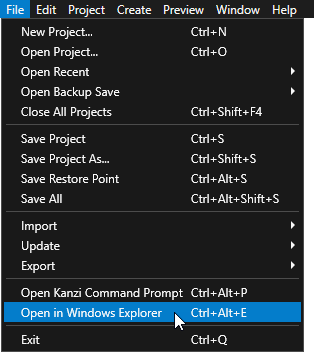
- In Visual Studio in the Solution Explorer right-click the Hello_world project and select Set as StartUp Project.
- In Visual Studio open the hello_world.cpp file.
Your application class uses the ExampleApplication
class which simplifies application development by handling the registration of the required modules.#include <kanzi/kanzi.hpp>
using namespace kanzi;
class HelloWorld : public ExampleApplication
- Include the log.hpp header file to use the logger to print to the Kanzi debug console:
#include <kanzi/core/log/log.hpp>
- When you place a function inside the
onProjectLoaded()
Kanzi calls the function after it loads your application.virtual void onProjectLoaded() KZ_OVERRIDE
{
// Prints Hello world! to the Kanzi debug console.
kzLogInfo(KZ_LOG_CATEGORY_GENERIC, ("Hello world!"));
}
- In Visual Studio select one of the solution configurations for your version of Visual Studio and run your application.
For example, if you are still developing your application, select the GL_vs2015_Debug configuration. If you want to create a production version of your Kanzi application, select one of the available release configurations.
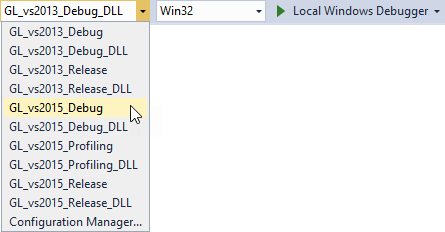
Function kzLogInfo
prints Hello world! to the Kanzi debug console.
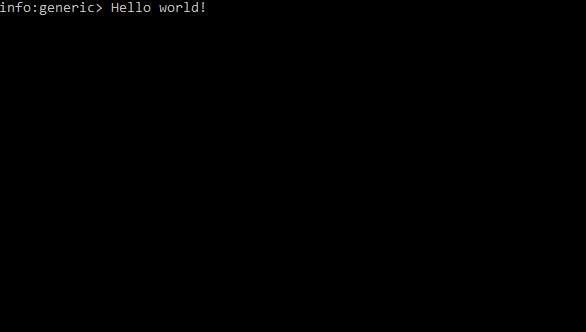
When in the debug mode, along with the Kanzi debug console, your Kanzi application is shown in the Kanzi player. Since by default a new Kanzi Studio project contains only a scene with a camera and a directional light, your application shows an empty scene.
This is what your hello_world.cpp looks like when you complete this step.
#include <kanzi/kanzi.hpp>
#include <kanzi/core/log/log.hpp>
using namespace kanzi;
class HelloWorld : public ExampleApplication
{
public:
virtual void onConfigure(ApplicationProperties& configuration) KZ_OVERRIDE
{
configuration.binaryName = "hello_world.kzb.cfg";
}
virtual void onProjectLoaded() KZ_OVERRIDE
{
// Project file was loaded from a kzb file.
kzLogInfo(KZ_LOG_CATEGORY_GENERIC, ("Hello world!"));
// Add initialization code here.
}
};
Application* createApplication()
{
return new HelloWorld;
}
< INTRODUCTION
NEXT STEP >
See also
API reference
Open topic with navigation